The hackin' page exhibits in the background the digits on the right.
These are an array (of arrays) of 0s and 1s that are updated in a zig-zag fashion,
starting from the bottom-right, proceeding inward and ending at the top-left.
Each row of numbers, however, shuffles the priority of these numbers so that
it appears somewhat random, all the while maintaining a visual diagonal refresh.
What follows are the steps to make something like this in After Effects and the JavaScript code used therein.
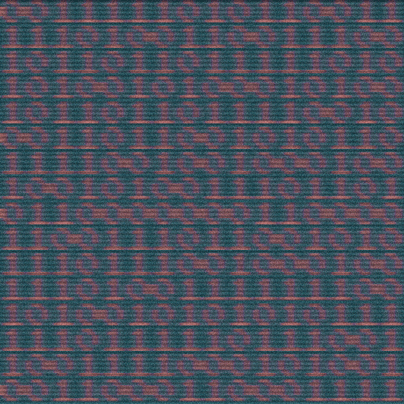
the setup
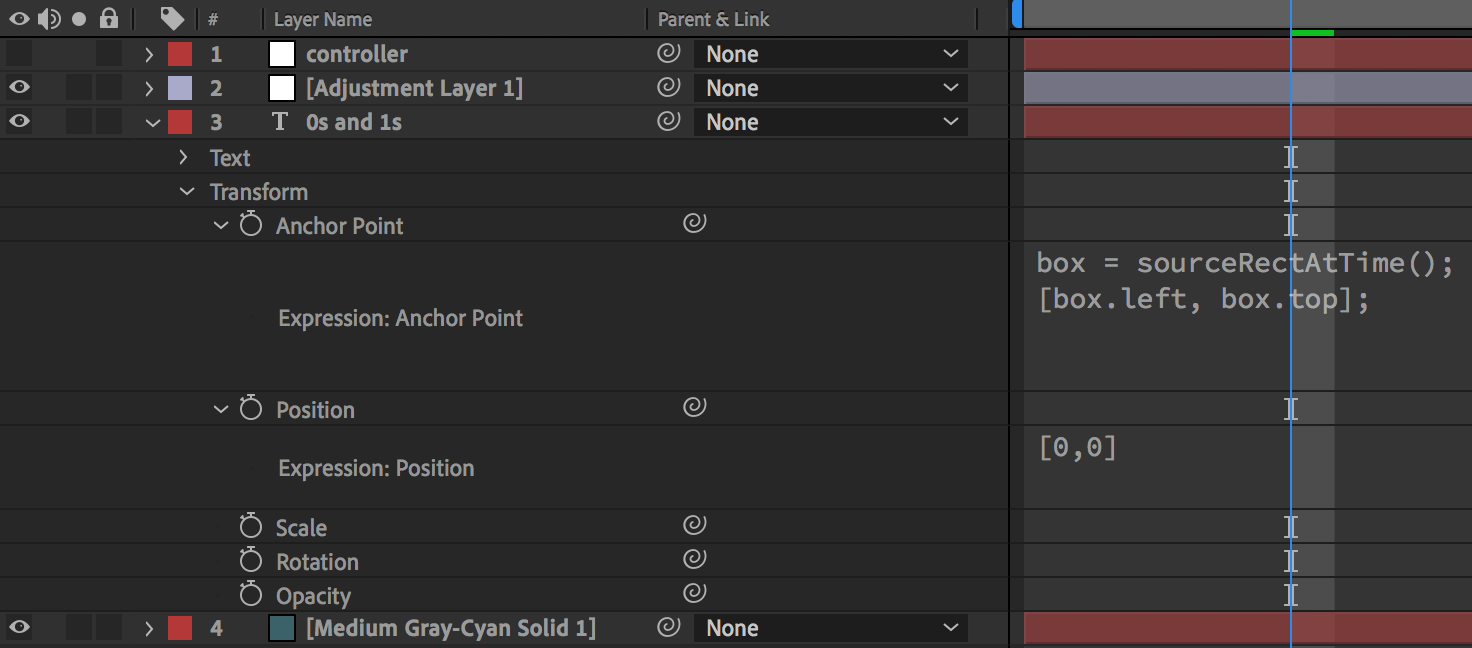
Here are the four layers used: the background cyan color, the actual digits themselves, an adjustment layer for graphical effects, and a layer that holds variables used in expressions that I don't want to manually change every time I test something.
The two expressions in the text layer are small, but they ensure that the text element begins and ends at the top-left corner of the page, despite whatever changes I may make to the font type and size.
The controller layer contains two sliders.
The period slider determines how often we begin another screen refresh and start updating the numbers from the bottom-right again. Here, this operation is performed every half-second.
The numRows slider determines both the width and height of the matrix. I elected to dismiss the case in which you might want more rows than columns or vice versa. I just wanted a perfect square of numbers. That's all I'm asking for. Just a good ol' perfect square. In the final product, I set this to 32.
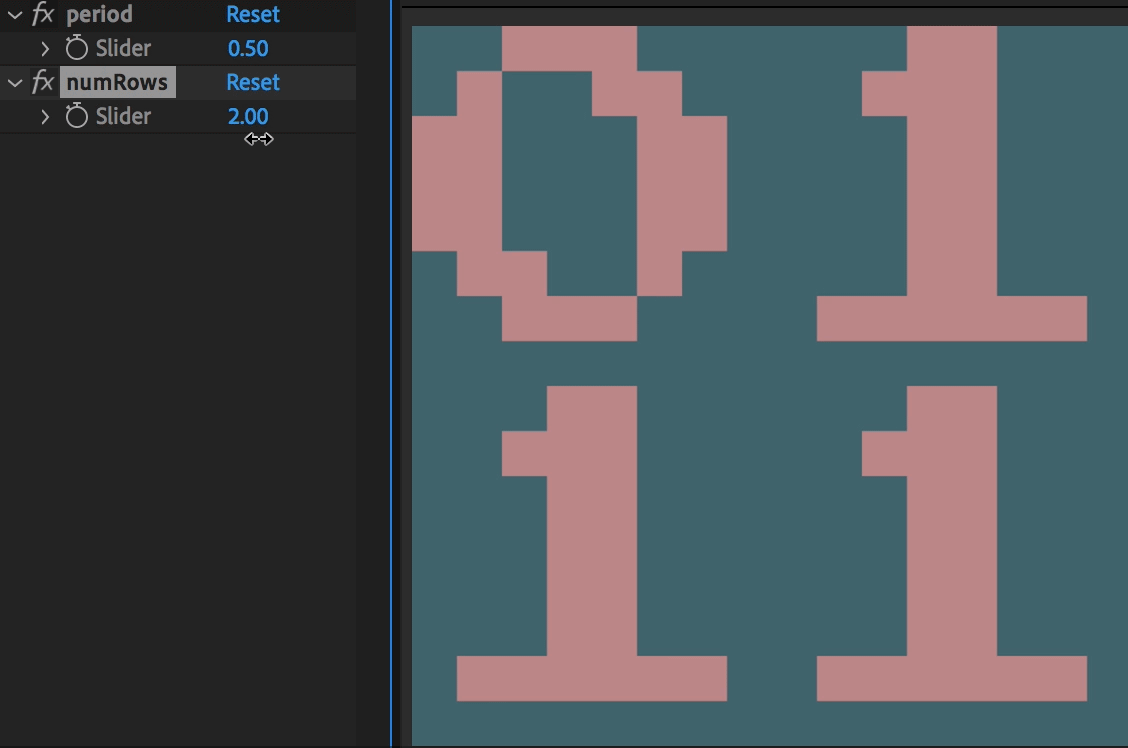
the code (enough chitchat!)
var period = thisComp.layer("controller").effect("period")("Slider");
var numRows = thisComp.layer("controller").effect("numRows")("Slider");
/* this 2D array is for reference only. Below this comment, I make the matrix dynamic.
const matToZZOrder = [
[ 0, 1, 5, 6, 14, 15, 27, 28 ],
[ 2, 4, 7, 13, 16, 26, 29, 42 ],
[ 3, 8, 12, 17, 25, 30, 41, 43 ],
[ 9, 11, 18, 24, 31, 40, 44, 53 ],
[ 10, 19, 23, 32, 39, 45, 52, 54 ],
[ 20, 22, 33, 38, 46, 51, 55, 60 ],
[ 21, 34, 37, 47, 50, 56, 59, 61 ],
[ 35, 36, 48, 49, 57, 58, 62, 63 ]
]; */
// Now I plan on reverse-engineering the matrix above
// with a variable width. Unfortunately I cannot vary
// height with this function. Oh well!
function ZigZagMatrix(n) {
mtx = [];
for (var i = 0; i < n; i++) {
mtx[i] = []; }
i=1; j=1;
for (var ct = 0; ct < n*n; ct++) {
mtx[i-1][i-1] = ct;
if ((i + j) % 2 == 0) {
// Even stripes
if (j < n) j ++;
else i+=2;
if (i > 1) i --;
} else {
// Odd stripes
if (i < n) i ++;
else j+=2;
if (j > 1) j --;
}
}
return mtx;
}
// Shuffle function!
function shuffle(array) {
var currentIndex = array.length, temporaryValue, randomIndex;
while (0 !== currentIndex) {
seedRandom(100276, true);
randomIndex = Math.floor(random() * currentIndex);
currentIndex -= 1;
//initate a pointer variable and swap em!
temporaryValue = array[currentIndex];
array[currentIndex] = array[randomIndex];
array[randomIndex] = temporaryValue;
}
return array;
}
// Store an appropriately-sized matrix
var zzMatrix = ZigZagMatrix(numRows);
// shuffle this matrix.
for (i = 0; i < numRows; i++) {
zzMatrix[i] = shuffle(zzMatrix[i]);
}
// Make the string
total = numRows*numRows;
str = "";
for (i = 0; i < numRows; i++) {
for (j = 0; j < numRows; j++) {
seed = Math.floor(time/period + zzMatrix[i][j]/total) + zzMatrix[i][j]*2100;
seedRandom(seed, true);
str += Math.round(random(0,1));
}
str += "\n";
}
//new, fancy AE2020 text stuff making me cum over here
text.sourceText.style.setFontSize(thisComp.width/numRows)
.setLeading(thisComp.width/numRows)
.setText(str);